2 min to read
간결한 본문 화살표 함수
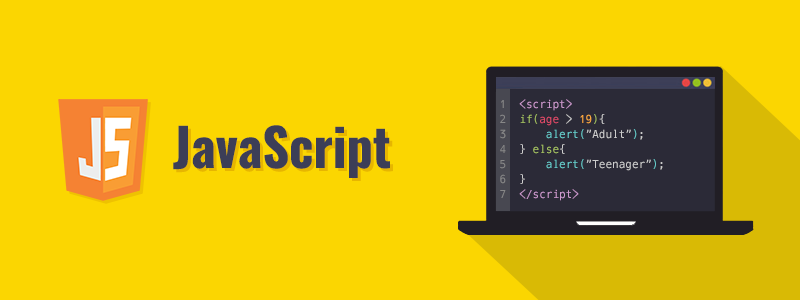
간결한 본문 화살표 함수(Consise body arrow functions)
JavaScript의 Concise body arrow functions는 하나의 표현식으로 이루어진 익명 함수를 정의하는 축약된 문법을 제공합니다. 이는 함수를 더 간결하고 표현력 있게 작성할 수 있는 방법을 제공합니다.
let add = function( a, b){
return a + b;
}
let subtract = ( a , b) => a - b;
console.log(add(2,2)); // output:4
console.log(subtract(3,4)); // output: -1
간결한 본문 화살표 함수의 이점
- 가독성: 읽기 쉽고 집중적인 표현이 가능
- 암시적 반환: 단일 표현식이 자동 반환되므로 명시적 return 문이 필요하지 않음
- 의도의 명확성: 함수 논리가 간단하고 한 줄로 표현될 수 있는 경우 유용함.
간결한 본문 화살표 함수 규칙
- 함수에 표현식이 하나만 있는 경우 return문이 필요 없이 값이 자동 반환됨.
const averageTwo = (x,y) => (x+y) /2; console.log(averageTwo(5,9)); // output : 7
- 함수가 하나의 인수만 사용하는 경우 인수 주위 괄호 생략가능
const sqaure = x => x * x; console.log(sqaure(5)); //output:25
- 함수에 인수가 없으면 빈 괄호쌍을 포함해야 함.
const hello = () console.log("Hello, World!"); console.log(Hello();) // output: Hello, World!
- 함수 본문이 여러 줄의 코드로 복잡한 경우 블록 본문 구문을 사용해야 함.
const absoluteValue = x => { if( x > 0){ return x; } else{ return -x; } }; console.log(absoluteValue(-5)); // output: 5
- 화살표 함수는 ‘this’,’arguments’,’super’,’new.target’ 키워드 사용을 지원하지 않음.
const obj ={ name: "Ada Lovelace", greeting : () => console.log('Hello, my name is ${ this.name}') }; obj.greet(); // output Hello, my name is undefined
- 화살표 함수는 객체 생성자로 사용할 수 없음. 객체 생성자를 호출하려면 ‘new’ 키워드를 사용해야함. ‘new’ 키워드와 함께 화살표 함수 사용시 에러가 발생.
const person = (name) => { this.name = name; } const john = new Person("John Doe"); // output: TypeError: Person is not a constructor